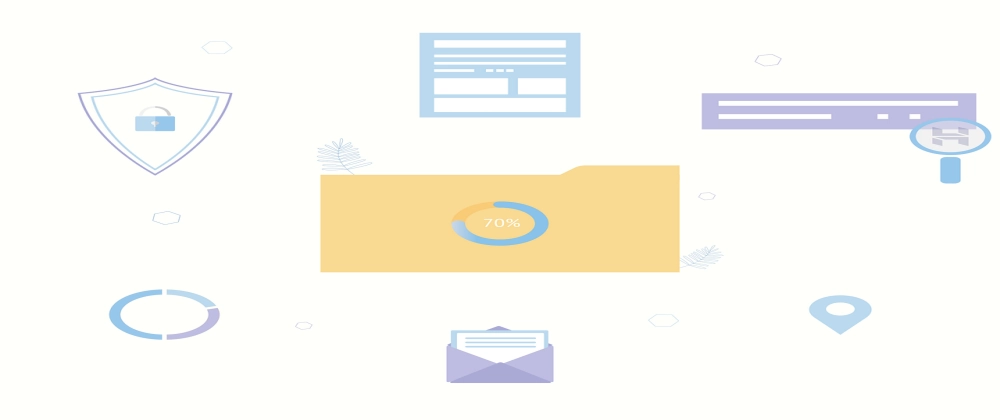
This post originally appeared on Michal's Corner
This guide is directed toward folks seeking instructions on generating documentation using their AsyncAPI files. Event-driven APIs are not the same as the synchronous APIs you usually document with OpenAPI or GraphQL. Many people use AsyncAPI now, and it is time to provide the community with a guide that shows what options the community has to render documentation on the client side.
Oh, if you are still not sure about client or server side rendering, then you can read all about it here Website rendering for beginners (shameless plug ).
I will cover the usage for:
1{
2 "asyncapi": "2.4.0",
3 "info": {
4 "title": "Account Service",
5 "version": "1.0.0",
6 "description": "This service is in charge of processing user signups"
7 },
8 "channels": {
9 "user/signedup": {
10 "subscribe": {
11 "message": {
12 "$ref": "#/components/messages/UserSignedUp"
13 }
14 }
15 }
16 },
17 "components": {
18 "messages": {
19 "UserSignedUp": {
20 "payload": {
21 "type": "object",
22 "properties": {
23 "displayName": {
24 "type": "string",
25 "description": "Name of the user"
26 },
27 "email": {
28 "type": "string",
29 "format": "email",
30 "description": "Email of the user"
31 }
32 }
33 }
34 }
35 }
36 }
37}
All usage examples from this article are available to check on the asyncapi-docs-rendering-examples repository.
React
If you wish to render documentation from your AsyncAPI file in React application, then you need to use the AsyncAPI React component.
npm install --save @asyncapi/react-component@next
1import React from "react";
2import ReactDOM from "react-dom";
3
4import AsyncApiComponent from "@asyncapi/react-component";
5import "@asyncapi/react-component/styles/default.min.css";
6
7import { specMock } from "./testDoc";
8
9const rootElement = document.getElementById("root");
10ReactDOM.render(<AsyncApiComponent schema={specMock} />, rootElement);
Here we can see that the React AsyncAPI component is imported on line 4.
If you are happy with AsyncAPI styling then you need to import their CSS pattern with:
import "@asyncapi/react-component/styles/default.min.css";
Line 7 is where the sample AsyncAPI file is imported.
Vue
If you wish to generate documentation from your AsyncAPI file in a Vue application, you need to use the AsyncApiStandalone bundle.
The given instructions are for Vue 2.x.
npm install --save @asyncapi/react-component@next
1<template>
2 <div ref="asyncapi"></div>
3</template>
4
5<script>
6import AsyncApiStandalone from '@asyncapi/react-component/browser/standalone';
7
8// AsyncAPI specification, fetched or pasted.
9const schema =
10'{"asyncapi":"2.4.0","info":{"title":"Account Service","version":"1.0.0","description":"This service is in charge of processing user signups"},"channels":{"user/signedup":{"subscribe":{"message":{"$ref":"#/components/messages/UserSignedUp"}}}},"components":{"messages":{"UserSignedUp":{"payload":{"type":"object","properties":{"displayName":{"type":"string","description":"Name of the user"},"email":{"type":"string","format":"email","description":"Email of the user"}}}}}}}';
11
12const config = {}; // Configuration for component. This same as for normal React component.
13
14export default {
15 name: 'AsyncApiComponent',
16 props: {
17 msg: String
18 },
19 mounted() {
20 const container = this.$refs.asyncapi;
21 AsyncApiStandalone.render({ schema, config }, container);
22 }
23}
24</script>
25
26<style scope src="@/assets/asyncapi.min.css"></style>
As you can see on line 6, you need to import the AsyncApiStandalone bundle with command
import AsyncApiStandalone from '@asyncapi/react-component/browser/standalone';
node_modules/@asyncapi/react-component/style/default.min.css
.
Copy that file and then paste it into your assets folder. I renamed mine asyncapi.min.css.
Then, you can import this in your main.js file with
import './assets/asyncapi.min.css'
You can also import it at the end of the App.vue file with:
<style scope src='./assets/asyncapi.min.css'></style>
Second way; import styles from @asyncapi/react-component
package:
<style scope src='@asyncapi/react-component/style/default.min.css'></style>
Web Components
To generate documentation from your AsyncAPI file, you can use it as an element of an HTML webpage or as a web component in any other web framework you choose. You can do this by using web-react-components.
1<script src="https://unpkg.com/@asyncapi/web-component@1.0.0-next.39/lib/asyncapi-web-component.js" defer></script>
2
3<asyncapi-component
4 schema='{"asyncapi":"2.4.0","info":{"title":"Account Service","version":"1.0.0","description":"This service is in charge of processing user signups"},"channels":{"user/signedup":{"subscribe":{"message":{"$ref":"#/components/messages/UserSignedUp"}}}},"components":{"messages":{"UserSignedUp":{"payload":{"type":"object","properties":{"displayName":{"type":"string","description":"Name of the user"},"email":{"type":"string","format":"email","description":"Email of the user"}}}}}}}'
5
6 config='{"show": {"sidebar": true}}'
7
8 cssImportPath="https://unpkg.com/@asyncapi/react-component@1.0.0-next.39/styles/default.min.css">
9</asyncapi-component>
<script src="https://unpkg.com/@webcomponents/webcomponentsjs@2.5.0/webcomponents-bundle.js"></script>
Standalone Bundle
If you want to render documentation from your AsyncAPI file without the use of any framework but with just an HTML webpage then you will need the Standalone bundle.
<link rel="stylesheet" href="https://unpkg.com/@asyncapi/react-component@1.0.0-next.39/styles/default.min.css">
1 <div id="asyncapi"></div>
2
3 <script src="https://unpkg.com/@asyncapi/react-component@1.0.0-next.39/browser/standalone/index.js"></script>
4 <script>
5 AsyncApiStandalone.render({
6 schema: '{"asyncapi":"2.4.0","info":{"title":"Account Service","version":"1.0.0","description":"This service is in charge of processing user signups"},"channels":{"user/signedup":{"subscribe":{"message":{"$ref":"#/components/messages/UserSignedUp"}}}},"components":{"messages":{"UserSignedUp":{"payload":{"type":"object","properties":{"displayName":{"type":"string","description":"Name of the user"},"email":{"type":"string","format":"email","description":"Email of the user"}}}}}}}'
7 ,
8 config: {
9 show: {
10 sidebar: true,
11 }
12 },
13 }, document.getElementById('asyncapi'));
14 </script>
This fetches everything required from the bundle
<script src="https://unpkg.com/@asyncapi/react-component@1.0.0-next.39/browser/standalone/index.js"></script>
There is one more way to configure the AsyncAPI component. You can do it through config props; the same as for the normal React component.
My Web Component and Standalone Bundle usage examples have
config='{"show": {"sidebar": true}}'
which turns on the sidebar, but if you change it to false then your rendered document will not have that sidebar. Whatever works best for you.
I almost forgot.
AsyncAPI Standalone Bundle can be used in Angular 2+. Please check the official docs if that is something that you may need.
This was only a sample of what could be done. AsyncAPI can do a lot more. Check out the AsyncAPI documentation for more information on how to amend the sample so it will meet your expectations.
I hope you liked this quick guide about the AsyncAPI client side document generation.